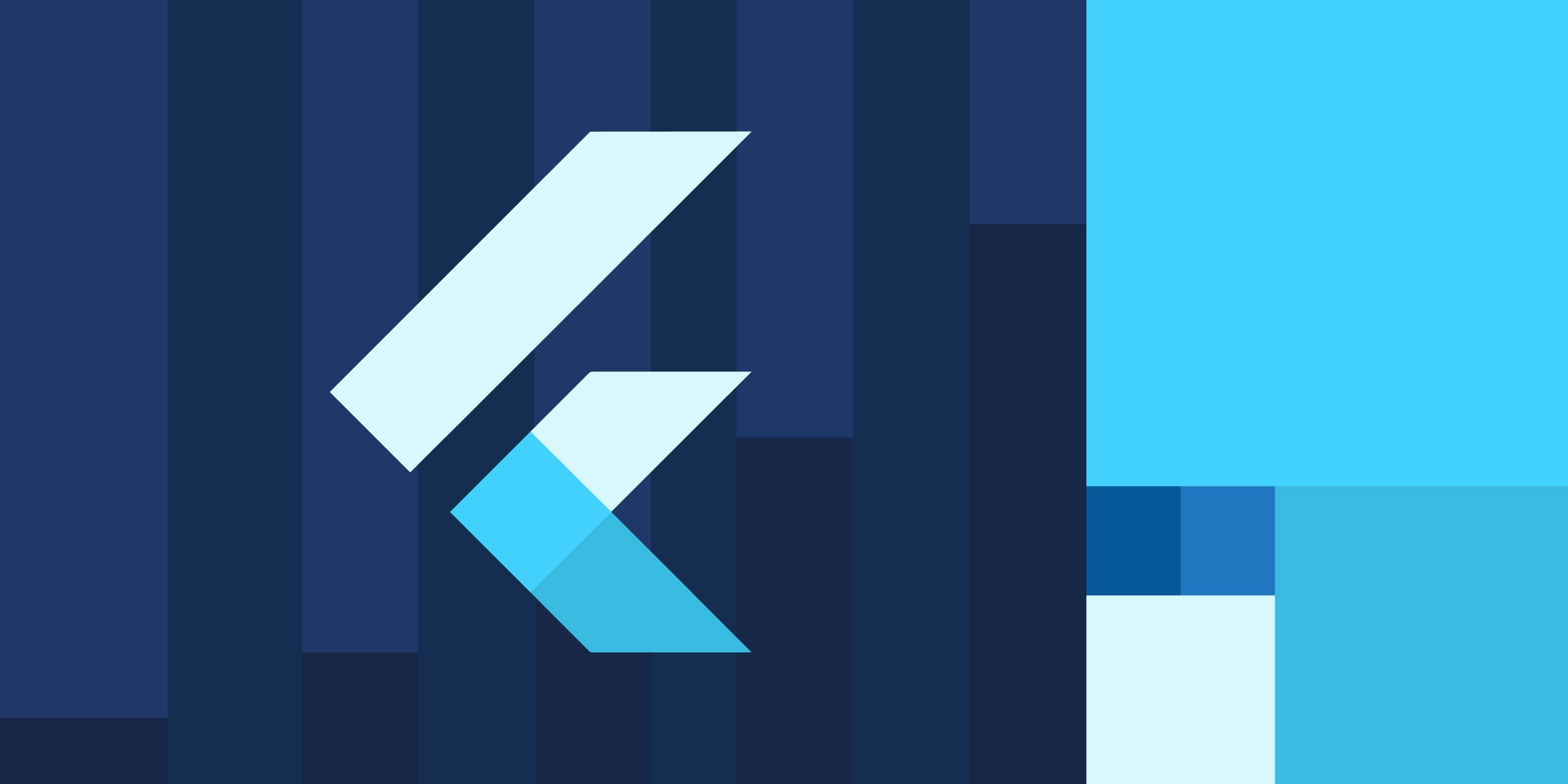
var data = {
"loading": true,
"items": [],
};
var data = {
"loading": true,
"items": null
};
doSomething() {
if(!data["loading"]) {
print(items.length); // Whoopsie!
}
}
enum RemoteState { initial, loading, empty, success, error }
class RemoteModel {
RemoteState state;
}
doSomething(RemoteModel model) {
switch(model.state) {
case RemoteState.initial:
// Handles error
break;
case RemoteState.loading:
// Handles error
break;
case RemoteState.success:
// Handles error
break;
case RemoteState.error:
// Handles error
break;
// Forgets to handle empty. Cries tears of sadness.
}
}
abstract class RemoteState {}
class RemoteStateInitial extends RemoteState{}
class RemoteStateLoading extends RemoteState {}
class RemoteStateEmpty extends RemoteState {}
class RemoteStateSuccess extends RemoteState {}
class RemoteStateError extends RemoteState {}
doSomething(RemoteState state) {
if(state is RemoteStateInitial) {
// Handles initial
}
if(state is RemoteStateEmpty) {
// Handles empty
}
if(state is RemoteStateSuccess) {
// Handles success
}
// Forgets to handle error & loading. Cries tears of sadness.
}
@freezed
abstract class RemoteState<T> with _$RemoteState<T> {
const factory RemoteState.initial() = _Initial<T>;
const factory RemoteState.loading() = _Loading<T>;
const factory RemoteState.success(T value) = _Success<T>;
const factory RemoteState.empty() = _Empty<T>;
const factory RemoteState.error([String message]) = _Error<T>;
}
// A class far, far away...
class RemoteModel {}
// Now when we handle our data model,
// we can properly represent the list of things
// with methods that represent async request state
doSomething(RemoteState<RemoteModel> state) {
counterState.when(
initial: () => { /* Handle initial */ },
empty: () => { /* Handle empty */ },
success: () => { /* Handle success */ },
)
}
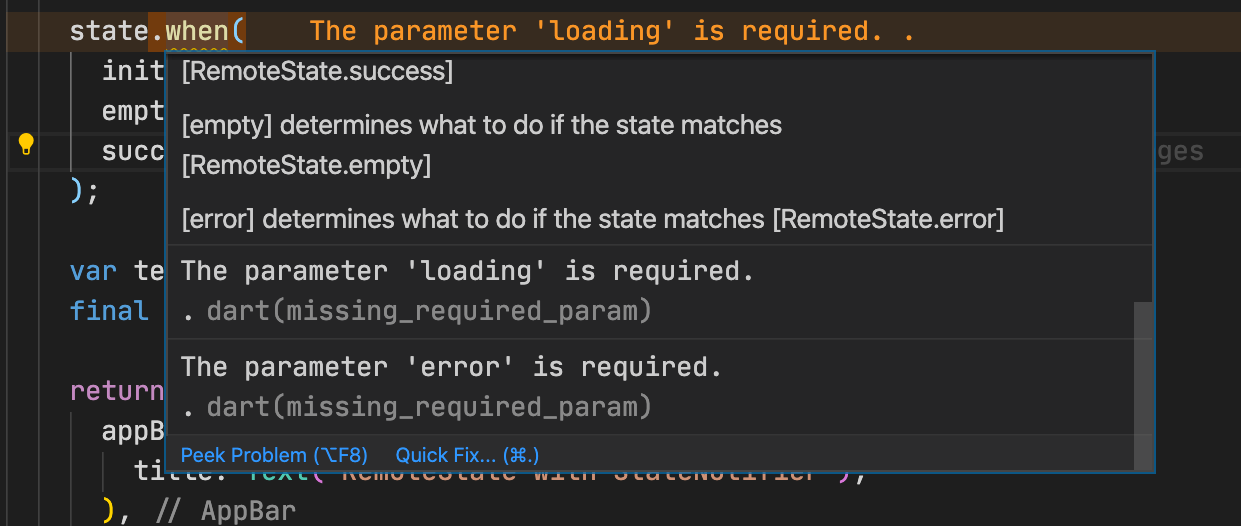
If you really want some joy, watch the original, maybe NSFW [video](https://www.youtube.com/watch?v=_QhuBIkPXn0)
If you really want some joy, watch the original, maybe NSFW [video](https://www.youtube.com/watch?v=_QhuBIkPXn0)
This article was originally published at https://chimon.hashnode.dev/slaying-a-ui...